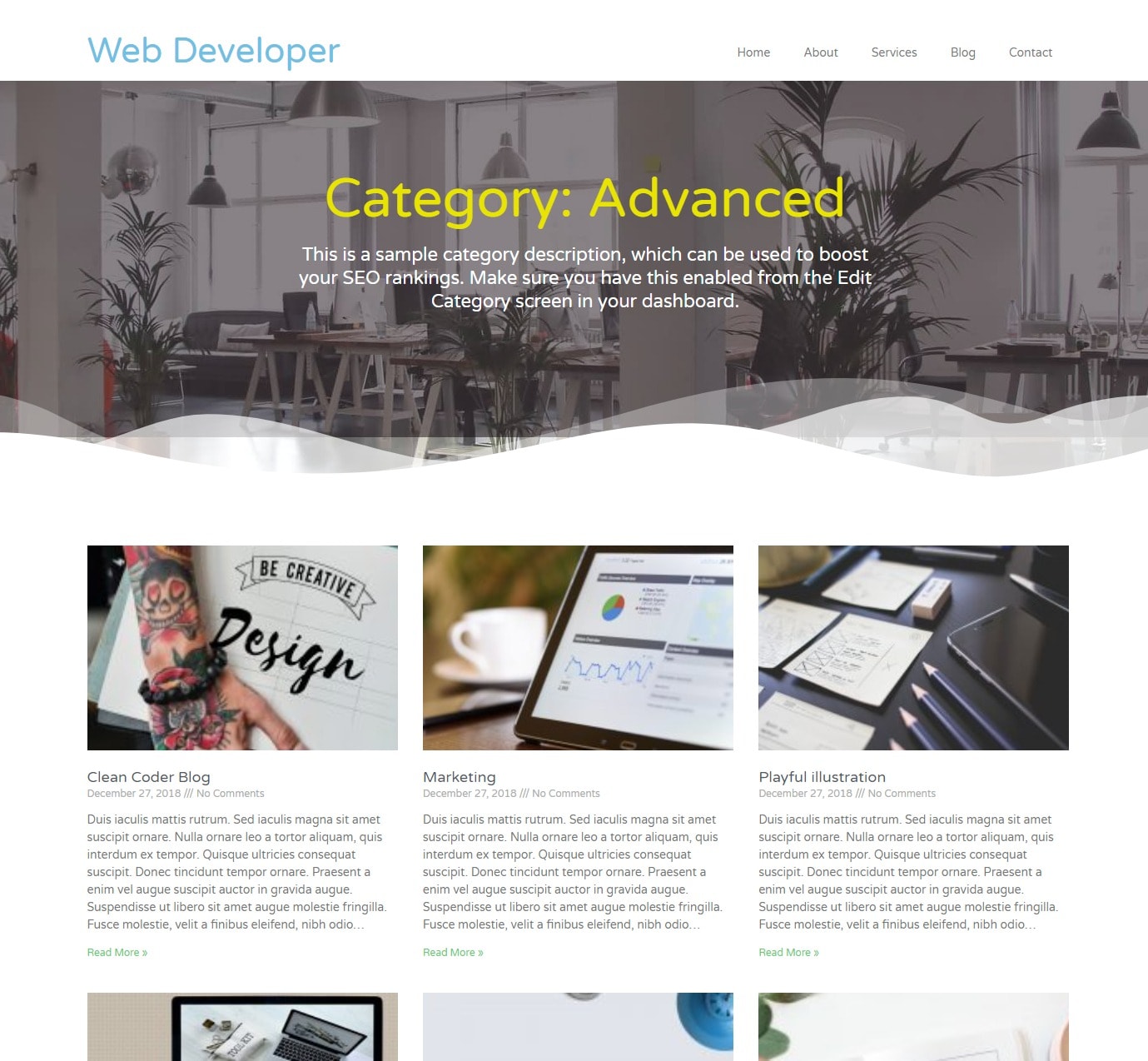

I build a category archive layout with Elementor theme builder. I create page header section and display the category name & description. I want to upload the category image from category’s add/edit page and add it to page header section as a background image. I tested it with 2 methods. You can use anyone.
- Method 1: Simple custom PHP codes (no extra plugin)
- Method 2: Advanced Custom Fields plugin
Minimum requirements
1. Elementor
2. Elementor Pro
Method 1: Simple custom PHP codes (no extra plugin)
By default there have no image upload option for categories. You need some extra codes or plugin for it. I am adding a image upload field in category add/edit form. So user can upload the custom image to individual category. Open your functions.php file and add the following scripts:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 |
//* Load media uploader js file add_action( 'admin_enqueue_scripts', 'paulc_admin_enqueue_scripts' ); function paulc_admin_enqueue_scripts() { $screen = get_current_screen(); $screen_id = $screen ? $screen->id : ''; // Edit category pages if ( in_array( $screen_id, array( 'edit-category' ) ) ) { wp_enqueue_media(); wp_enqueue_script( 'cat-media-uploader', get_stylesheet_directory_uri() . '/js/media.uploader.js', array(), CHILD_THEME_VERSION, true ); wp_localize_script( 'cat-media-uploader', 'MU', array( 'title' => __( "Choose an image", "theme-prefix" ), 'button_text' => __( "Use image", "theme-prefix" ), 'placeholder' => get_stylesheet_directory_uri() . "/images/placeholder.png" ) ); } } /** * Adding media uploader button in Add & Edit form * Also saving the data when creating or updating a category */ add_action( 'admin_init', 'paulc_category_featured_image_field' ); function paulc_category_featured_image_field() { add_action( 'category_add_form_fields', 'paulc_category_add_form_fields' ); add_action( 'category_edit_form_fields', 'paulc_category_edit_form_fields', 10 ); add_action( 'created_term', 'paulc_save_category_form_fields', 10, 3 ); add_action( 'edit_term', 'paulc_save_category_form_fields', 10, 3 ); } /** * Callback function. Adding placeholder image with upload button */ function paulc_category_add_form_fields() { ?> <div class="form-field"> <label><?php _e( 'Featured Image', 'theme-prefix' ); ?></label> <div id="cat_feat_img" style="padding-top: 10px;"><img src="<?php echo get_stylesheet_directory_uri() ;?>/images/placeholder.png" width="220px" height="220px" /></div> <div style="padding-top: 10px;"> <input type="hidden" id="cat_feat_img_id" name="cat_feat_img_id" /> <button type="button" class="upload_image_button button"><?php _e( 'Upload/Add image', 'theme-prefix' ); ?></button> <button type="button" class="remove_image_button button"><?php _e( 'Remove image', 'theme-prefix' ); ?></button> </div> <div class="clear"></div> </div> <?php } /** * Edit term's featured image * * @param mixed $term Term (category) being edited */ function paulc_category_edit_form_fields( $term ) { $cat_feat_img_id = absint( get_term_meta( $term->term_id, 'cat_feat_img_id', true ) ); if ( $cat_feat_img_id ) { $image = wp_get_attachment_thumb_url( $cat_feat_img_id ); } else { $image = get_stylesheet_directory_uri() . '/images/placeholder.png'; } ?> <tr class="form-field"> <th scope="row" valign="top"><label><?php _e( 'Featured Image', 'theme-prefix' ); ?></label></th> <td> <div id="cat_feat_img" style="margin-bottom: 10px;"><img src="<?php echo esc_url( $image ); ?>" width="220px" height="220px" /></div> <div> <input type="hidden" id="cat_feat_img_id" name="cat_feat_img_id" value="<?php echo $cat_feat_img_id; ?>" /> <button type="button" class="upload_image_button button"><?php _e( 'Upload/Add image', 'theme-prefix' ); ?></button> <button type="button" class="remove_image_button button"><?php _e( 'Remove image', 'theme-prefix' ); ?></button> </div> <div class="clear"></div> </td> </tr> <?php } /** * Saving data * * @param mixed $term_id Term ID being saved * @param mixed $tt_id * @param string $taxonomy */ function paulc_save_category_form_fields( $term_id, $tt_id = '', $taxonomy = '' ) { if ( isset( $_POST['cat_feat_img_id'] ) && '' !== $taxonomy ) { update_term_meta( $term_id, 'cat_feat_img_id', absint( $_POST['cat_feat_img_id'] ) ); } } //* Adding the column name add_filter( 'manage_edit-category_columns', 'paulc_category_columns' ); function paulc_category_columns( $paulc_category_columns ) { array_shift( $paulc_category_columns ); $new_columns = array(); $new_columns['cb'] = ''; $new_columns['cat_image_thumb'] = __( 'Image', 'theme-prefix' ); return array_merge( $new_columns, $paulc_category_columns ); } //* Add image thumb at Image column add_filter('manage_category_custom_column', 'paulc_category_column_image', 10, 3 ); function paulc_category_column_image( $arg1, $column_name, $term_id ) { if ( $column_name == 'cat_image_thumb' ) { $cat_feat_img_id = absint( get_term_meta( $term_id, 'cat_feat_img_id', true ) ); if ( $cat_feat_img_id ) { $image = wp_get_attachment_thumb_url( $cat_feat_img_id ); } else { $image = get_stylesheet_directory_uri() . '/images/placeholder.png'; } return sprintf( '<img src="%s" width="60px" height="60px" />', esc_url( $image ) ); } } add_action( 'wp_head', 'paulc_add_og_image_category_page' ); function paulc_add_og_image_category_page() { if( ! is_category() ) { return ''; } $term_id = 0; $image = ''; $queried_object = get_queried_object(); if ( is_object( $queried_object ) && isset( $queried_object->term_id ) ) { $term_id = $queried_object->term_id; $cat_feat_img_id = absint( get_term_meta( $term_id, 'cat_feat_img_id', true ) ); if ( $cat_feat_img_id ) { $image = wp_get_attachment_url( $cat_feat_img_id ); echo '<meta property="og:image" content="' . $image . '" />' . "\n"; } } return ''; } |
2. Creates Media Uploader JS file
Creates a new file “media.uploader.js” and put in your theme’s “js” folder. This JS file will open the media uploader form in modal window. Here is the full code of this JS file:
The rest of the content is available for Pro members. Click on the PAY NOW button to enable access to this content.
$10.99Pay Now
* Payment is non-refundable.
Paid members will be able to login below to see the restricted content.
Trista DeVries (verified owner) –
This was a HUGE help with something I’d been fighting with for a couple of days. I had some issues with the code presented above, but emailed and got support nearly instantly. Now it’s working perfectly. Wish I’d found this ages ago. I’ll be back to check here first the next time I have a complex (and slightly weird) problem. Don’t hesitate. Just buy the pro version to see the whole tutorial.
Kasper Andersen (verified owner) –
Thank you for doing this.
So far I can’t get it working though.
I get the category under dynamic tags and I have created and added a category image to the post, but I don’t get any image – not even the fallback image shows. Any ideas. I’m on Elementor 3.5.5 and Pro 3.6.2.
The stage page is here: https://kulturfesten.holycow.media
Dominik Rajdl (verified owner) –
Very simple and clear: Took me five minutes and works perfectly. Thank you very much!
yu cheng wang (verified owner) –
well written, clear and workable!