How do you add a custom post type in your Beaver Builder theme without any plugin? I want to create a custom post type “portfolio” to showcase my work. I used the following PHP scripts in Beaver Builder Child theme.
Register Custom Post Type “Portfolio”
Open the class-fl-child-theme.php file from bb-theme-child/classes folder. Add the following PHP snippet above the last curly bracket ‘}‘.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<?php // Do not include this line static public function register_custom_post_type_portfolio() { $portfolio_labels = array( 'name' => __( 'Portfolio', 'taxonomy general name', 'fl-builder'), 'singular_name' => __( 'Portfolio Item', 'fl-builder'), 'search_items' => __( 'Search Portfolio Items', 'fl-builder'), 'all_items' => __( 'Portfolio', 'fl-builder'), 'parent_item' => __( 'Parent Portfolio Item', 'fl-builder'), 'edit_item' => __( 'Edit Portfolio Item', 'fl-builder'), 'update_item' => __( 'Update Portfolio Item', 'fl-builder'), 'add_new_item' => __( 'Add New Portfolio Item', 'fl-builder') ); $portfolio_slug = 'portfolio-item'; $args = array( 'labels' => $portfolio_labels, 'rewrite' => array('slug' => $portfolio_slug,'with_front' => false), 'singular_label' => __('Portfolio', 'fl-builder'), 'public' => true, 'publicly_queryable' => true, 'show_ui' => true, 'hierarchical' => false, 'menu_position' => 9, 'menu_icon' => 'dashicons-art', 'supports' => array( 'title', 'editor', 'thumbnail' ) ); register_post_type( 'portfolio' , $args ); } |
Now open the functions.php file of BB child theme and add this single line at end of the file:
1 2 3 |
<?php //* Do not include this line add_action( 'init', 'FLChildTheme::register_custom_post_type_portfolio' ); |
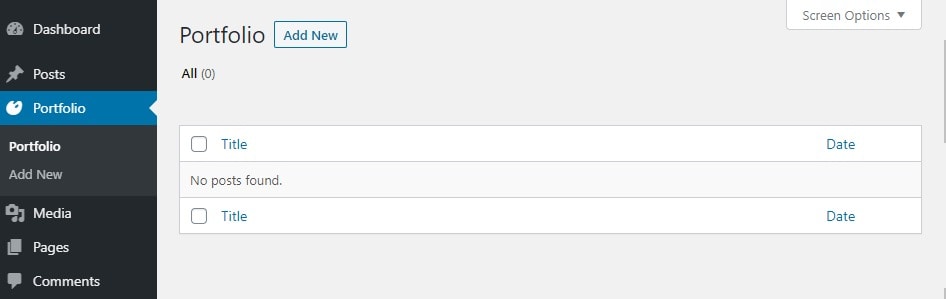
After this you can do extra things. You can register the custom taxonomy for portfolio category. I used the following the steps.
Register Taxonomy “portfolio-cat”
Again open the class-fl-child-theme.php file and add this small PHP snippet there.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<?php //* Do not include this line static public function register_taxonomy_portfolio_cat() { $category_labels = array( 'name' => __( 'Categories', 'fl-builder' ), 'singular_name' => __( 'Category', 'fl-builder' ), 'search_items' => __( 'Search Portfolio Categories', 'fl-builder' ), 'all_items' => __( 'All Portfolio Categories', 'fl-builder' ), 'parent_item' => __( 'Parent Portfolio Category', 'fl-builder' ), 'edit_item' => __( 'Edit Portfolio Category', 'fl-builder' ), 'update_item' => __( 'Update Portfolio Category', 'fl-builder' ), 'add_new_item' => __( 'Add New Portfolio Category', 'fl-builder' ), 'menu_name' => __( 'Categories', 'fl-builder' ) ); $permalink_slug = 'portfolio-cat'; register_taxonomy("portfolio-cat", array("portfolio"), array( 'hierarchical' => true, 'labels' => $category_labels, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => $permalink_slug ) ) ); } |
Later you will add this single PHP code into the functions.php file.
1 2 3 |
<?php //* Do not include this line add_action( 'init', 'FLChildTheme::register_taxonomy_portfolio_cat' ); |

Here is the complete code of class-fl-child-theme.php file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
<?php /** * Helper class for child theme functions. * * @class FLChildTheme */ final class FLChildTheme { /** * Enqueues scripts and styles. * * @return void */ static public function enqueue_scripts() { wp_enqueue_style( 'fl-child-theme', FL_CHILD_THEME_URL . '/style.css' ); } /** * Register custom post type. New CPT: portfolio * * @return void */ static public function register_custom_post_type_portfolio() { $portfolio_labels = array( 'name' => __( 'Portfolio', 'taxonomy general name', 'fl-builder'), 'singular_name' => __( 'Portfolio Item', 'fl-builder'), 'search_items' => __( 'Search Portfolio Items', 'fl-builder'), 'all_items' => __( 'Portfolio', 'fl-builder'), 'parent_item' => __( 'Parent Portfolio Item', 'fl-builder'), 'edit_item' => __( 'Edit Portfolio Item', 'fl-builder'), 'update_item' => __( 'Update Portfolio Item', 'fl-builder'), 'add_new_item' => __( 'Add New Portfolio Item', 'fl-builder') ); $portfolio_slug = 'portfolio-item'; $args = array( 'labels' => $portfolio_labels, 'rewrite' => array('slug' => $portfolio_slug,'with_front' => false), 'singular_label' => __('Portfolio', 'fl-builder'), 'public' => true, 'publicly_queryable' => true, 'show_ui' => true, 'hierarchical' => false, 'menu_position' => 9, 'menu_icon' => 'dashicons-art', 'supports' => array( 'title', 'editor', 'thumbnail' ) ); register_post_type( 'portfolio' , $args ); } /** * Register custom taxonomy for portfolio post type. * * @return void */ static public function register_taxonomy_portfolio_cat() { $category_labels = array( 'name' => __( 'Categories', 'fl-builder' ), 'singular_name' => __( 'Category', 'fl-builder' ), 'search_items' => __( 'Search Portfolio Categories', 'fl-builder' ), 'all_items' => __( 'All Portfolio Categories', 'fl-builder' ), 'parent_item' => __( 'Parent Portfolio Category', 'fl-builder' ), 'edit_item' => __( 'Edit Portfolio Category', 'fl-builder' ), 'update_item' => __( 'Update Portfolio Category', 'fl-builder' ), 'add_new_item' => __( 'Add New Portfolio Category', 'fl-builder' ), 'menu_name' => __( 'Categories', 'fl-builder' ) ); $permalink_slug = 'portfolio-cat'; register_taxonomy("portfolio-cat", array("portfolio"), array( 'hierarchical' => true, 'labels' => $category_labels, 'show_ui' => true, 'query_var' => true, 'rewrite' => array( 'slug' => $permalink_slug ) ) ); } } |
Reset the Permalink Settings
We shall avoid the 404 error for single portfolio and taxonomy archive pages. Navigate to Dashboard -> Settings -> Permalink page and click on “Save Changes” button. It will flush the permalink structure and new permalinks of Portfolio items will work perfectly.
Leave a Reply